Blog
February 21, 2020
IBM i data is stored in Db2 databases. But what's the best way to leverage this data? That's what we break down in this blog. First, let's cover the basics.
What Is a Db2 Database?
Back to topA Db2 database is a relational database management system (RDBMS) from IBM. You can use a Db2 database to store and manage your IBM i data.
Why You Need to Modernize Your Db2 Database
Modernization is a popular topic in the IBM i community. And it extends to your Db2 databases. Here's how you need to modernize them:
- Through web forms/pages that allow for more dynamic and deeper displays of information in Db2 databases.
- By leveraging the web to make Db2 data available to a wider set of constituents, stakeholders, and customers.
In this blog, you'll learn how to create web-based applications written in PHP that can work with your IBM i data and provide CRUD functionality.
If you've not heard the term CRUD before, it stands for Create, Read, Update, and Delete.
Back to topDoes Db2 Use SQL?
Back to topDb2 databases use SQL as the language to access data.
Db2 SQL Commands
Back to topA CRUD-based application can use basic Db2 SQL commands to retrieve, update, and manipulate information in Db2 databases.
How to Unleash Your Db2 Database With PHP
The following are the three most common approaches for implementing a PHP, CRUD-based application.
- Custom PHP scripts.
- phpGrid.
- MVC middleware/frameworks, specifically Laminas (formerly Zend Framework).
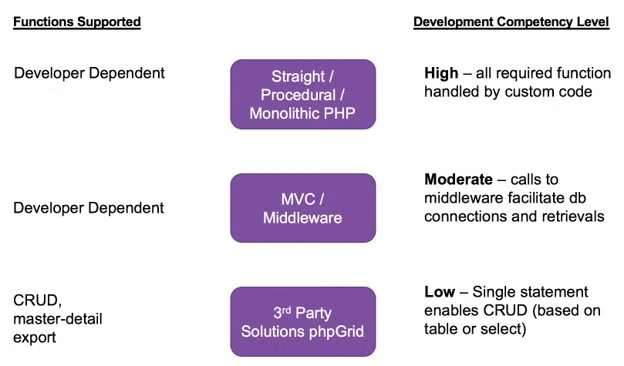
1. Custom PHP Scripts
The first approach to talk about is developing your own custom PHP scripts. For this approach, you need to:
- Develop separate scripts that handle each of the four functions of CRUD.
- Link the scripts, as needed, via the html 'href' attribute.
Typically, the scripts manage the logic, data presentation, and user-input gathering via HTML. So, when you use this approach, the business logic will not be separate from the data representation.
You could use one monolothic script. Or you could separate the main functions into separate scripts. For example, using CRUD commands, you could write a:
- Create script that presents an HTML form that collects data and adds a record to the database/table.
- Read a script that provides a form that can be used to input search criteria that selects the requested records. This script could be used as the "input" for the Update and Delete functions. This is done by using an 'href' against the key field of each returned record.
- Update script that selects a specific record or form, and populates it with the field-data from the selected record. An <Update> function would perform an SQL UPDATE function, programmatically from PHP, using the data from the update form.
- Delete script that deletes the selected record using a SQL DELETE statement. To avoid errors, it’s a good idea to include a deletion confirmation step in your delete scripts.
Pros and Cons of Custom PHP Scripts
PHP scripts aren't the fastest approach. You'll need to develop custom scripts for each function. No framework would be used. This means that functions — such as connecting to the data store — would need to be implemented several times within the scripts.
This leads to high levels of code repetition. It also can create a difficult situation for maintenance down the road. Imagine having 50 instances and having to change one of these functions?
Scripts can get complex, too. That's because they need to provide forms that are appealing to users and representative of data formats. This requires the retrieval of data-attribute information. And if the data attributes change, you need to change the various forms used to display and manipulate data. And this might possibly be in multiple places throughout the scripts.
Third Party Applications – PHPgrid
You could also use a third-party solution, such as PHPgrid, to unleash your Db2 databases in PHP.
The Pros and Cons of PHPgrid
PHPgrid removes much of the minutia you’ll have to manage when you develop custom scripts. In addition, it can be easier to provide a relatively clean display of your data using basic CRUD functionality. For example:
<!DOCTYPE html>
<?php
use phpgrid\C_DataGrid;
require_once("phpGrid/conf.php");
$dg = new C_DataGrid("SELECT * FROM SP_CUST","CUST_ID","SP_CUST");
$dg -> display();
?>
The above code snippet is a complete PHP script that provides the following data grid:
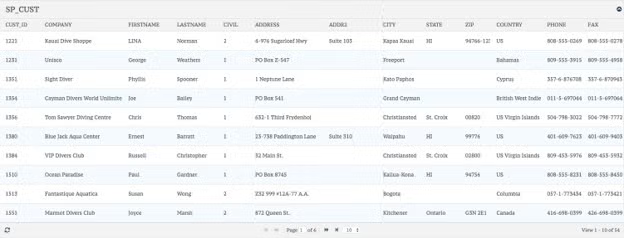
And, it is relatively simple to add additional CRUD functionality. All you need to do is add the following lines to your script:
- Search: $dg -> enable_search(true);
- Create, Update, Delete: $dg -> enable_edit("FORM");
- Master/Detail:
$sdg = new C_DataGrid("SELECT * FROM ORDER", "CUST_ID", "ORDERS");
$dg->set_masterdetail($sdg, "CUST_ID");
The disadvantage of using PHPgrid is that it can be challenging to evolve or scale the application to deliver new functionality. For example, it could be difficult to add a function that uses the records retrieved via PHPgrid as input to an RPG call (via the iToolkit/XMLService).
MVC Middleware/Frameworks — Specifically Laminas
You could also leverage a PHP framework and middleware, such as the Laminas Project (formerly Zend Framework) and its corresponding middleware solution, Mezzio (formerly Expressive).
If you have been around PHP development for a while, you probably know all about frameworks. If you haven't been exposed to frameworks, don't worry. Here are some definitions that will help:
- A framework provides a platform for streamlining the development of web applications.
- Middleware are applications that sit between applications to pre-process incoming requests and post-process outgoing responses.
MVC stands for Model, View, Controller. It is a design paradigm or pattern for managing the requests of web-based applications by separating the:
- Application’s model or business logic that govern data manipulation.
- View or user interface.
- Controller, which uses the model to process all data received and displayed by the user interface, or view, including GET calls, POST data, and uploaded files.
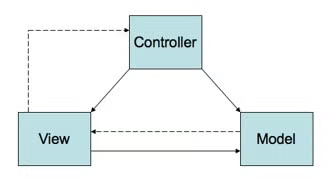
It’s worth noting that you would use either MVC or middleware to manage your web application’s requests.
Back to topWhy Use Frameworks For Db2 Databases?
You can simplify and streamline the development of web-based, PHP applications that run on the IBM i platform by using frameworks. They eliminate the need to develop the same solution or write the same code over and over again. Frameworks also establish connections between your application and data sources.
Most frameworks, including Laminas, adhere to the Model-View-Controller (MVC) design pattern. The concept behind MVC is to isolate the business logic of an application from user interface considerations. By isolating the business logic from the user interface, it becomes easier to modify either without affecting the other.
Laminas
The Laminas Project is the new name of Zend Framework!
Zend by Perforce continues to be a major influencer and developer of the Laminas Project. But the framework is now open source from Linux Foundation as the Laminas Project.
It is important to keep in mind that Laminas is not a new framework but rather a continuation of the Zend Framework. This framework has been continuously developed by an extensive community over the last 15 years — and downloaded 400 million times. Additionally, it brings extensibility and testability to PHP applications.
Mezzio
If you prefer to use middleware, you can use Mezzio. It is a subproject of Laminas that provides middleware for building PHP applications that use the PSR-7 (HTTP Messages) and PSR-15 (HTTP Request Handlers) specifications. It also provides a variety of routing and templating options.
PSR stands for PHP Standard Recommendation. PSRs are interfaces or APIs produced by the PHP Framework Interop Group (PHP-FIG) for the purpose of standardizing common application concerns within PHP frameworks and applications. Middleware such as Mezzio is driven, in part, through implementation of various PSRs.
When it comes to developing CRUD applications, the PSRs that matter include:
- PSR-7 (HTTP Messages): APIs that define HTTP messages, including requests, responses, URIs, and file uploads.
- PSR-15 (Request Handlers and Middlware): APIs that define request handlers and middleware that consume PSR-7 server-side requests and produce PSR-7 responses. PSR-15 enables the separation of common requests and the processing of responses from applications’ business logic.
- PSR-17 (HTTP Factories): APIs for defining factories, allowing middleware to be written agnostic of PSR-7 implementations. This allows changing the underlying PSR-7 implementation when needed.
So why would we want to use middleware? Put simply, middleware:
- Simplifies the creation of custom workflows with every endpoint.
- Speeds response times for users and applications by creating only the objects needed for the specific request.
- Simplifies application development, testing, and maintenance by supporting abstraction that helps to build more modular applications — and simplify CI/CD workflows, even in distributed environments.
Frameworks such as Laminas rely on Composer to install and update applications’ various components. When the application structure is first created, a directory structure such as the following is created:
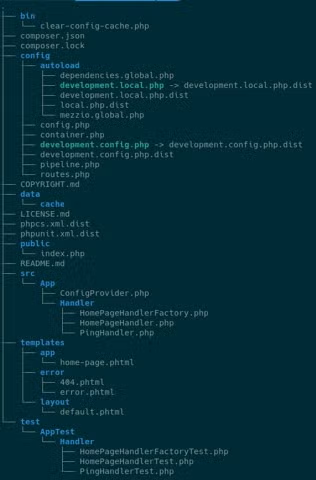
Structure items include:
- Handler: processes (handles) a request and produces a response as defined by PSR-7.
- Interface: defines required methods (functions).
- Factory: an object that creates other objects and provides what they need to complete their work.
- ConfigProvider: determines configuration for the application module.
- Table: deals with data access/db2.
- Customer: represents a single customer.
- CreateCustomerFromArray: a class that creates a customer instance from an array of values.
Creating Middleware with Mezzio
At a very high level, to create a Mezzio application, you will first use Composer to create the project:
composer create-project mezzio/mezzio-skeleton mezzio
After answering a few questions regarding interfaces for routing and templating, you can start writing your middleware. Generally, the default responses suffice, except when it comes to an engine. You should specify one because the default is to omit it.
Get an example of middleware code >>
Back to topUnleash Your Db2 Database With PHP
You need to modernize your Db2 database. And the best way to do it is with PHP.
You'll be able to unlock data siloes, so that you make use of historic and current data. You can also deliver new services that people take advantage of using their desktops and mobile devices.
Regardless of which method you choose to develop your PHP apps — custom PHP scripts, phpGrid, frameworks such as Laminas, or middleware such as Mezzio — Zend can help.
Our PHP and Laminas experts can help you with your projects by providing:
- PHP and framework training and certification.
- Professional services that can help with development, deployment, CI/CD, and more using PHP, Zend Server, Laminas, and its projects including Mezzio.
- Laminas Enterprise Support to ensure your applications meet your mission-critical uptime requirements.
- ZendPHP Enterprise runtimes that come with mission-critical support.
- Zend Server which is an industry-leading PHP application server with comprehensive tools including real-time debugging and mission-critical support.
Questions?
Additional Resources
- 101 Guide - Developing Web Applications with PHP
- Blog - Monitoring PHP on IBM i
- Blog - What Is a PHP Function?
- Blog - How to Use Db2 Services for PHP Apps on IBM i